The last of the list with Python
Python is known for making it easy to write beautiful, small code. Today’s pro tip is about how to get the last element of a list using this language \o/
You can watch below or continue reading:
Coming from other languages like Java or C to Python, it’s normal to bring a bit of an accent to your codes and want to do something more or less like this to get the last element of a list:
my_list = [1, 2, 3, 4, 5]
item_index = len(my_list) - 1
print(item_index)
# 4
last_one = my_list[item_index]
print(last_one)
# 5
Use the len()
size function to get the length of the list and subtract 1
to get the index of the last element in that list. And that’s ok! It works. However, Python presents a more elegant way of doing this, see:
last_one = my_list[-1]
print(last_one)
# 5
Even though it may seem like this, it’s not magic! From the Python documentation:
If i or j is negative, the index is relative to the end of sequence s:
len(s) + i
orlen(s) + j
is substituted. But note that-0
is still0
.
In a simpler way, Raymond Hettinger explains in this tweet with code:
#python tip: How to support negative indexing:
— Raymond Hettinger (@raymondh) 20 de dezembro de 2017
def __getitem__(self, i):
if i < 0:
i += len(self)
if i < 0 or i >= len(self):
raise IndexError
...
The implementation is actually more complex than this, but what happens in general terms: the __getitem__
method is invoked when we call my_list[-1]
receiving the list itself (self
) and the negative index (i
) then adds this index with the size of the list and returns the updated index value. Interestingly, this was the same thing I did on the first example but it is already implemented by default.
And a little detail, the same can be done for strings!
word = 'yes'
print(word[-1])
# s
Dope, right? Now just use negative index in your codes too 😉
Links
- For more details on string slicing, check out this informal Introduction to Python
- Reference to the special case of negative indexes in the
__getitem__
method in the Python documentation
Special thanks
To Mário Sérgio, Diego Ponciano and Paulo Haddad for some of the links in this post!
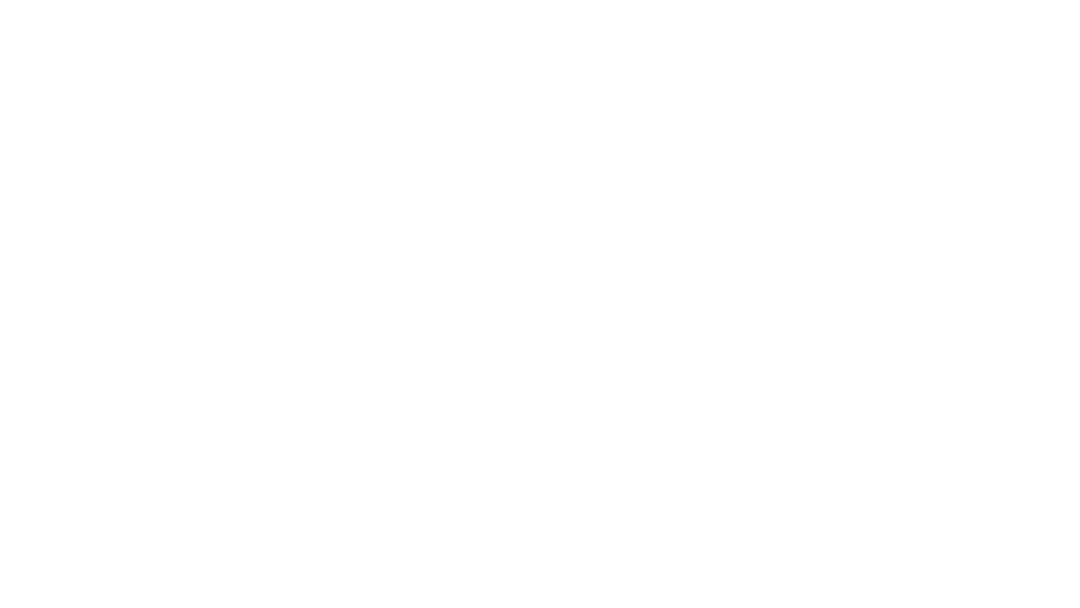